Ultimate Guide to React JS: Building Modern Web Applications
Hosted by Jeff Smith
Tweet ShareReact JS, developed by Facebook, has revolutionized the way web applications are built. Its component-based architecture, virtual DOM, and declarative approach have made it the go-to choice for developers looking to create fast, efficient, and maintainable web applications. In this comprehensive React JS guide, we'll take you on a journey through the world of React JS, from the fundamentals to advanced techniques, best practices, and real-world applications.
What is React JS? React JS, often simply referred to as React, is an open-source JavaScript library for building user interfaces. It was developed by Facebook and released in 2013. React's main goal is to enable developers to create highly efficient and dynamic web applications that can seamlessly update and render user interfaces as data changes.
React follows a component-based architecture, where UIs are broken down into small, reusable components. These components encapsulate the logic and presentation of a specific part of the user interface, making it easier to manage, test, and maintain complex applications.
Key Features and Benefits React's popularity stems from its powerful features and numerous benefits:
Virtual DOM: React uses a virtual DOM to optimize the updating process, resulting in faster rendering and improved performance.
Declarative Syntax: React's declarative syntax allows developers to describe how the UI should look based on the application's state, making code more readable and predictable.
Component Reusability: Components are self-contained units that can be reused throughout the application, leading to more maintainable and modular code.
Unidirectional Data Flow: React follows a unidirectional data flow, which means data flows in one direction from parent to child components, making it easier to track changes.
JSX: JSX is a syntax extension that allows developers to write HTML-like code within JavaScript, enhancing the readability of UI code.
Why Choose React for Web Development? Choosing React for web development comes with several advantages:
Rich Ecosystem: React has a vast ecosystem of libraries, tools, and extensions that enhance its capabilities and facilitate development.
Large Community: React has a strong and active community of developers, which means you'll find ample resources, tutorials, and solutions to common problems.
Fast Rendering: Thanks to the virtual DOM and efficient diffing algorithm, React's rendering process is highly optimized and provides excellent performance.
SEO-Friendly: React's server-side rendering (SSR) capabilities enable better search engine optimization, resulting in improved search rankings.
Setting Up Your Development Environment
Installing Node.js and npm Before you start building React applications, you'll need Node.js and npm (Node Package Manager) installed on your computer. Node.js allows you to run JavaScript code on the server side, while npm manages packages and dependencies for your projects.
To install Node.js and npm, visit the official Node.js website and download the installer for your operating system. Once installed, you can verify the installation by opening a terminal and running the following commands:
node -v npm -v You should see the versions of Node.js and npm displayed in the terminal.
Creating Your First React App React provides a tool called create-react-app that sets up a new React project with all the necessary dependencies and configurations. To create your first React app, follow these steps:
Open a terminal and run the following command to install create-react-app globally:
npm install -g create-react-app Once the installation is complete, navigate to the directory where you want to create your React app and run the following command:
create-react-app my-app Replace my-app with the name you want for your app. This command will create a new directory with the app's name and set up the project structure.
Navigate to the app's directory: sh Copy code cd my-app To start the development server and see your app in the browser, run the following command: sh Copy code npm start This will start the development server and automatically open your app in your default web browser.
Understanding Project Structure The project structure created by create-react-app is designed to help you organize your code efficiently. Here's an overview of the main directories and files:
src: This directory contains the main source code for your application. index.js: The entry point of your application, where you render your root component. App.js: The default root component created by create-react-app. You can modify this component to build your app. public: This directory contains public assets, such as the index.html file that serves as the template for your app's HTML structure. node_modules: This directory contains the dependencies installed for your project. package.json: This file contains metadata about your project, as well as a list of dependencies and scripts. 3. React Components Introduction to Components At the heart of React development are components. Components are the building blocks of your user interface, and they encapsulate the behavior and appearance of various parts of your application. Components can be simple, such as a button, or complex, like a form or a chat widget.
There are two main types of components in React:
Functional Components: These are JavaScript functions that return JSX (JavaScript XML) to define the component's UI. Functional components are stateless and primarily responsible for rendering content.
Class Components: Class components are ES6 classes that extend the React.Component class. They have additional features, such as local state and lifecycle methods, making them suitable for managing complex logic and state.
Creating Functional Components Functional components are a fundamental concept in React. They are simple to create and use. Here's an example of a functional component:
import React from 'react';
function Welcome(props) { return
Hello, {props.name}
; }export default Welcome; In this example, we define a functional component called Welcome that takes a name prop and displays a greeting. You can use this component in other parts of your application by importing it and including it in your JSX.
Managing State with Class Components Class components in React have an additional feature: they can have local state. State allows components to store and manage data that can change over time, leading to dynamic and interactive user interfaces.
Here's an example of a class component that manages a counter:
import React, { Component } from 'react';
class Counter extends Component { constructor(props) { super(props); this.state = { count: 0 }; }
increment = () => { this.setState({ count: this.state.count + 1 }); };
render() { return (
Count: {this.state.count}
export default Counter; In this example, the Counter component has a count property in its state, which is initially set to 0. The increment method is used to update the state when the button is clicked, and the current count is displayed in the JSX.
Props and Prop Types Props (short for properties) are a way to pass data from parent to child component in React. They allow components to be dynamic and reusable. Here's an example of a component that uses props:
import React from 'react'; import PropTypes from 'prop-types';
function Greeting(props) { return
Hello, {props.name}!
; }Greeting.propTypes = { name: PropTypes.string.isRequired, };
export default Greeting; In this example, the Greeting component accepts a name prop, which is used to customize the greeting message. We also use PropTypes to define the expected prop types and indicate that name is required.
Component Lifecycle Class components have a lifecycle that consists of various phases, each with its own lifecycle methods. These methods allow you to perform tasks at specific points in the component's existence, such as when it's first mounted to the DOM or when it's about to be unmounted.
Some important lifecycle methods include:
componentDidMount(): This method is called after the component has been rendered to the DOM. It's often used for tasks like data fetching.
componentDidUpdate(prevProps, prevState): This method is called after a component's props or state change. It's useful for responding to changes in data.
componentWillUnmount(): This method is called just before a component is removed from the DOM. It's used for cleanup tasks like unsubscribing from event listeners.
- React and JSX Introducing JSX JSX, or JavaScript XML, is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript files. JSX makes it easier to define the structure of your UI components in a format that closely resembles HTML.
JSX Expressions JSX allows you to embed JavaScript expressions within curly braces {}. This enables dynamic content rendering and the use of variables and functions.
Conditional Rendering JSX also allows you to perform conditional rendering using JavaScript's conditional operators like if and the ternary operator ?. Here's an example:
function Greeting(props) { const isLoggedIn = props.isLoggedIn;
return (
Welcome back!
) : (Please sign in.
)}Lists and Keys When rendering lists of elements in React, it's common to use the map() method to create a new array of JSX elements. Each element in the array should have a unique key prop to help React identify which items have changed, added, or removed when updating the list.
function ListItems(props) { const items = props.items;
return (
-
{items.map((item) => (
- {item.text} ))}
Styling in React Styling in React can be done using traditional CSS, but it's also common to use CSS-in-JS solutions like styled-components or inline styles. Here's an example of applying inline styles:
const buttonStyle = { backgroundColor: 'blue', color: 'white', padding: '10px 20px', border: 'none', };
function MyButton() { return ; } In this example, the buttonStyle object is used to define the button's styles, which are then applied using the style attribute.
- State Management with Hooks React introduced Hooks in version 16.8, which allow functional components to manage state and side effects. Prior to Hooks, state management was primarily handled by class components.
Introducing Hooks Hooks are functions that enable you to "hook into" React state and lifecycle features from functional components. Some of the most commonly used Hooks include:
useState(): This Hook allows functional components to manage state. It returns an array with the current state value and a function to update it.
useEffect(): This Hook handles side effects in functional components. You can perform data fetching, subscriptions, or manually change the DOM in a useEffect function.
useContext(): This Hook enables functional components to access the context of a parent component, providing a way to share values like themes or authentication status.
useReducer(): This Hook is often used for more complex state management, especially when state transitions follow specific patterns or logic.
useState for Managing State The useState Hook allows functional components to manage state by providing a way to declare and initialize state variables.
import React, { useState } from 'react';
function Counter() { // Declare a state variable named "count" with an initial value of 0 const [count, setCount] = useState(0);
return (
Count: {count}
useEffect for Side Effects The useEffect Hook is used to perform side effects in functional components. Side effects can include data fetching, manually changing the DOM, or subscribing to external data sources.
import React, { useState, useEffect } from 'react';
function DataFetcher() { const [data, setData] = useState(null);
useEffect(() => { // Perform data fetching or other side effects here fetch('https://api.example.com/data') .then((response) => response.json()) .then((data) => setData(data)); }, []); // The empty array [] means this effect runs once after the initial render
return (
Data: {data}
:Loading...
}Custom Hooks Custom Hooks are a way to reuse stateful logic in functional components. They are regular JavaScript functions that start with the word "use" and can call other Hooks if needed.
Conclusion
In this extensive React JS tutorial guide, we've journeyed through the world of React JS, covering everything from its fundamental concepts to advanced techniques and real-world application development. React has proven itself as a powerful library for building modern web applications, and understanding its core principles is essential for any web developer. Cronj's team of React experts can provide valuable insights, develop tailored reactjs development services india solutions, and ensure the success of your web applications.
Comments
Attendees (1)
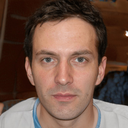