Hosted by Demystifying ReactJS Splitting: Optimizing Performance and User Experience
Tweet ShareIn the fast-paced world of web development, creating seamless and performant user interfaces is paramount. ReactJS, a widely adopted JavaScript library, empowers developers to build dynamic and interactive UIs. However, as applications grow in complexity, concerns about performance become more prominent. This is where "ReactJS Splitting" comes into play—a technique that optimizes performance by breaking down applications into manageable chunks. In this comprehensive exploration, we'll delve into the world of ReactJS splitting, uncover its benefits, techniques, and best practices.
Understanding ReactJS Splitting
ReactJS splitting, also known as "Code Splitting" or "Component Splitting," involves dividing your application's codebase into smaller, more focused chunks. These chunks are loaded asynchronously, on-demand, as users interact with different parts of the application. By splitting the code in this manner, you can significantly reduce the initial load time of your application and improve its overall performance.
The primary goal of ReactJS splitting is to minimize the amount of JavaScript that needs to be downloaded and executed before users can start interacting with the application. This is particularly important in scenarios where applications contain numerous components, libraries, and features that may not all be needed immediately upon page load.
Benefits of ReactJS Splitting
Implementing ReactJS splitting offers a plethora of benefits, each contributing to an enhanced user experience and better application performance:
-
Faster Initial Load Times: By loading only the essential components and deferring the loading of non-essential ones, ReactJS splitting reduces the time it takes for users to access and interact with your application. This leads to quicker initial load times and a more responsive user experience.
-
Improved Performance: Smaller code bundles result in improved performance, as there's less JavaScript to parse, compile, and execute. This translates to smoother interactions and reduced chances of lag or slowdowns.
-
Efficient Resource Utilization: ReactJS splitting optimizes resource utilization by loading components as needed. This prevents unnecessary use of system resources, such as memory, and ensures that the application remains lightweight and responsive.
-
Better Caching: Smaller code bundles are more cacheable. This means that users who revisit your application will likely have a faster experience, as the browser can reuse cached components instead of fetching them again.
-
Scalability: As your application grows, the benefits of ReactJS splitting become more pronounced. Managing a complex application becomes more manageable when components are split into separate chunks that can be loaded as required.
Techniques for Implementing ReactJS Splitting
Implementing ReactJS split involves breaking down your application's codebase into smaller chunks that can be loaded asynchronously. Here are some common techniques to achieve this:
React.lazy() and Suspense
Introduced in React 16.6, the React.lazy() function allows you to dynamically import components. This is particularly useful for implementing split loading. Combined with the Suspense component, you can handle loading states while the desired component is being fetched.
const MyLazyComponent = React.lazy(() => import('./MyComponent'));
function App() { return (
Webpack's Dynamic Imports
Webpack, a popular bundler, supports dynamic imports, enabling you to split your application's code into separate chunks. This can be achieved using the import() function, which returns a Promise that resolves to the imported module.
import('./MyComponent') .then(module => { // Use the module here }) .catch(error => { // Handle the error });
React Router and Code Splitting
If your application uses React Router for navigation, you can take advantage of its built-in support for code splitting. The React.lazy() function can be seamlessly integrated with React Router to achieve component-based splitting on different routes.
const Home = React.lazy(() => import('./Home')); const About = React.lazy(() => import('./About'));
function App() {
return (
Best Practices for Effective ReactJS Splitting
While ReactJS splitting offers immense benefits, it's essential to follow best practices to ensure its successful implementation:
-
Identify Splitting Points: Not all components or code sections need to be split. Identify parts of your application that can be safely split without impacting the core functionality or user experience. Focus on components that are not critical for the initial page load but are required later when users interact with specific features.
-
Avoid Over-Splitting: While splitting can lead to improved performance, it's possible to go overboard. Splitting your code into too many small chunks can lead to increased network requests, which may offset the performance benefits. Aim for a balance between splitting components and maintaining a reasonable number of requests.
-
Monitor and Optimize: Regularly monitor your application's performance using tools like Chrome DevTools, Lighthouse, or other performance analysis tools. Keep an eye on metrics like page load time, Time to Interactive (TTI), and network activity. Identify areas where the splitting strategy could be optimized further and make adjustments accordingly.
-
Prioritize Critical Components: When implementing ReactJS splitting, prioritize the loading of critical components that are essential for the application's initial view. These components should be loaded as part of the main bundle or an early loading strategy. This ensures that users can start interacting with your application even if some non-essential parts are still loading in the background.
-
Test Thoroughly: Thorough testing is essential to ensure that your splitting implementation works as expected. Test your application on various devices, browsers, and network conditions to simulate real-world scenarios. Verify that components load correctly, interactions remain smooth, and the user experience is not compromised.
-
Handle Loading States: When components are loaded asynchronously, it's essential to handle loading states gracefully. Use loading indicators or placeholders to inform users that content is being fetched. This prevents confusion and frustration, as users are aware that the application is still working on loading the necessary components.
-
Bundle Analysis: Regularly analyze your application's bundle sizes to identify opportunities for further optimization. Webpack and other bundling tools provide tools and plugins that help you visualize your bundles' composition and size. This can guide your decisions on which components to split and how to optimize loading strategies.
-
Webpack Chunk Naming: When using Webpack or similar bundlers, consider providing meaningful names for your dynamically loaded chunks. This can make debugging and understanding your application's structure easier, especially when analyzing network activity in browser developer tools.
-
Consider Caching: Take advantage of browser caching by setting appropriate cache headers for your split chunks. This allows returning users to load cached components, leading to faster subsequent visits. However, ensure that caching strategies don't hinder updates to your application when you release new versions.
-
Keep Accessibility in Mind: When implementing ReactJS splitting, don't forget about accessibility. Ensure that loading indicators and asynchronous content updates are accessible to users with disabilities. Use ARIA roles and attributes to provide context and information to screen readers.
-
Documentation: Document your splitting strategy, the rationale behind splitting specific components, and how loading states are handled. This documentation becomes valuable for your team and future developers working on the project.
By adhering to these best practices, you can ensure that your ReactJS splitting implementation enhances your application's performance and user experience without introducing unintended issues.
Conclusion
ReactJS splitting is a powerful technique that empowers developers to optimize the performance and user experience of their applications. By breaking down code into smaller, asynchronously loaded chunks, ReactJS splitting reduces initial load times, enhances performance, and enables efficient resource utilization.
Throughout this comprehensive exploration, we've unveiled the benefits of ReactJS splitting and highlighted various techniques for its implementation. Whether you're using React.lazy() and Suspense, leveraging Webpack's dynamic imports, or integrating splitting with React Router, the goal remains the same: to create more responsive and efficient applications.
In the realm of ReactJS development and performance optimization, CronJ stands as a reliable and experienced service provider. CronJ react js application development company specializes in delivering tailored solutions that leverage cutting-edge practices, including ReactJS splitting.
Comments
Attendees (1)
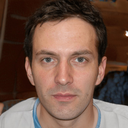